Digital Marketing
Difference Between Tuple And List in Python A full guide
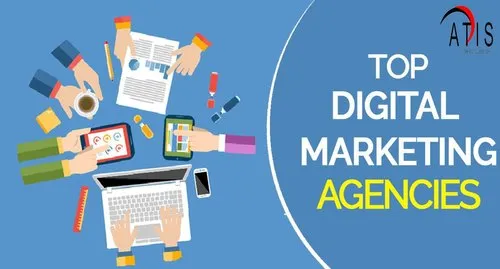
SEO Meta Description: Discover the difference between tuple and list in Python, and explore their unique features and use cases. This comprehensive article compares tuple and list, highlighting their syntax, mutability, performance, and when to use each data structure. Read on to gain a deeper understanding of tuple and list in Python.
Introduction
Python, a popular and versatile programming language, provides various data structures to store and manipulate data efficiently. Among these, two fundamental data structures are tuples and lists. Both tuples and lists serve as containers to hold multiple elements, but they differ in their properties and use cases. In this article, we will delve into the difference between tuple and list in Python, examining their characteristics and practical applications.
Difference Between Tuple and List
Syntax and Declaration
Tuples and lists are both ordered collections, but they have distinct syntax and declaration methods.
Tuple Syntax
A tuple is defined using parentheses, and its elements are separated by commas. Once a tuple is created, its elements cannot be modified or added, making tuples immutable.
Example:
python
Copy code
my_tuple = (1, 2, 3, ‘hello’, True)
List Syntax
On the other hand, a list is declared using square brackets, with elements separated by commas. Unlike tuples, lists are mutable, allowing elements to be modified, added, or removed after creation.
Example:
python
Copy code
my_list = [4, 5, 6, ‘world’, False]
Mutability
One of the most significant distinctions between tuples and lists is their mutability.
Tuple Mutability
Tuples are immutable, meaning their elements cannot be changed once they are created. This immutability ensures data integrity and is particularly useful when dealing with constant data that should not be modified.
List Mutability
In contrast, lists are mutable, enabling the addition, removal, or modification of elements after initialization. This feature makes lists highly flexible and suitable for situations where data needs to be updated frequently.
Performance
Performance is a critical aspect to consider when choosing between tuples and lists, especially for large datasets.
Tuple Performance
Tuples, being immutable, offer better performance than lists in certain scenarios. Since tuples cannot be changed, they have a smaller memory footprint and are generally faster to access and process.
List Performance
While lists may have slightly lower performance compared to tuples due to their mutability, they are still highly efficient for most use cases. The ability to modify lists in-place can sometimes outweigh the minor performance difference.
Use Cases
The choice between tuples and lists depends on the specific use case and the requirements of the program.
Tuple Use Cases
Tuples are best suited for scenarios where data needs to be constant and unchangeable. Some common use cases include:
- Storing coordinates (x, y) of points in a 2D plane.
- Defining keys for dictionary entries, as dictionaries require immutable keys.
List Use Cases
Lists are more versatile and find applications in situations that require dynamic data manipulation. Some typical use cases include:
- Maintaining a collection of user records in a database.
- Implementing a stack or queue data structure.
Indexing and Slicing
Both tuples and lists support indexing and slicing to access specific elements.
Indexing in Tuple and List
Indexing in both data structures is zero-based, meaning the first element is accessed using index 0, the second with index 1, and so on.
Example:
python
Copy code
my_tuple = (10, 20, 30, 40, 50)
my_list = [100, 200, 300, 400, 500]
print(my_tuple[0]) # Output: 10
print(my_list[2]) # Output: 300
Slicing in Tuple and List
Slicing allows you to extract a range of elements from a data structure.
Example:
python
Copy code
my_tuple = (1, 2, 3, 4, 5)
my_list = [10, 20, 30, 40, 50]
print(my_tuple[1:4]) # Output: (2, 3, 4)
print(my_list[:3]) # Output: [10, 20, 30]
Concatenation and Repetition
Both tuples and lists support concatenation and repetition operations.
Concatenation
Concatenation combines two or more tuples or lists to create a new collection.
Example:
python
Copy code
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
list1 = [10, 20, 30]
list2 = [40, 50, 60]
new_tuple = tuple1 + tuple2
new_list = list1 + list2
print(new_tuple) # Output: (1, 2, 3, 4, 5, 6)
print(new_list) # Output: [10, 20, 30, 40, 50, 60]
Repetition
Repetition duplicates a tuple or list multiple times.
Example:
python
Copy code
tuple1 = (1, 2)
list1 = [10, 20]
repeated_tuple = tuple1 * 3
repeated_list = list1 * 4
print(repeated_tuple) # Output: (1, 2, 1, 2, 1, 2)
print(repeated_list) # Output: [10, 20, 10, 20, 10, 20, 10, 20]
Membership Test
You can check if an element is present in a tuple or list using the “in” keyword.
Example:
python
Copy code
my_tuple = (1, 2, 3, 4, 5)
my_list = [10, 20, 30, 40, 50]
print(3 in my_tuple) # Output: True
print(25 in my_list) # Output: False
Built-in Functions
Python provides several built-in functions that can be used with both tuples and lists.
Common Built-in Functions
Some common functions applicable to both data structures include:
- len(): Returns the number of elements in the tuple or list.
- max(): Returns the maximum element in the tuple or list.
- min(): Returns the minimum element in the tuple or list.
Example:
python
Copy code
my_tuple = (10, 20, 30, 40, 50)
my_list = [100, 200, 300, 400, 500]
print(len(my_tuple)) # Output: 5
print(max(my_list)) # Output: 500
print(min(my_tuple)) # Output: 10
Converting Between Tuple and List
Python allows converting between tuples and lists using built-in functions.
Converting Tuple to List
You can use the list() function to convert a tuple to a list.
Example:
python
Copy code
my_tuple = (1, 2, 3, 4, 5)
my_list = list(my_tuple)
print(my_list) # Output: [1, 2, 3, 4, 5]
Converting List to Tuple
Similarly, you can use the tuple() function to convert a list to a tuple.
Example:
python
Copy code
my_list = [10, 20, 30, 40, 50]
my_tuple = tuple(my_list)
print(my_tuple) # Output: (10, 20, 30, 40, 50)
FAQs
FAQ 1: What is the primary difference between tuples and lists in Python? Answer: The main difference is that tuples are immutable, while lists are mutable. Once a tuple is created, its elements cannot be modified, whereas lists allow for changes in their elements after initialization.
FAQ 2: When should I use a tuple instead of a list? Answer: Tuples are best suited for situations where the data needs to remain constant and should not be modified. For example, tuples are commonly used to store fixed coordinates or keys for dictionaries.
FAQ 3: In what scenarios are lists preferred over tuples? Answer: Lists are preferred when you require a dynamic data structure that can be modified or updated during the program’s execution. Lists are commonly used to store collections of data that might change over time.
FAQ 4: Can I convert a tuple to a list or vice versa in Python? Answer: Yes, Python provides built-in functions to convert between tuples and lists. You can use list() to convert a tuple to a list and tuple() to convert a list to a tuple.
FAQ 5: What is the significance of mutability in data structures? Answer: Mutability impacts how data can be modified after its creation. Immutable data structures, like tuples, ensure data integrity and prevent unintended changes, while mutable data structures, like lists, offer flexibility in updating and manipulating data.
FAQ 6: Which data structure is more efficient in terms of performance? Answer: Tuples are generally more efficient in terms of performance due to their immutability, resulting in a smaller memory footprint and faster access times. However, the performance difference is often negligible for most use cases.
Conclusion
In conclusion, understanding the difference between tuple and list in Python is crucial for selecting the appropriate data structure for your specific programming needs. Tuples provide immutability and better performance, making them suitable for storing constant data, while lists offer mutability and greater flexibility, making them ideal for dynamic data manipulation. By considering the unique properties of tuples and lists, you can optimize your code and enhance its efficiency.
===========================================